Purpose
I had the requirement to create a form with, among many other fields, an email address field. Rather than use the usual validation model on the button component or a validation model on the output path, I decided to use javascript to implement validation directly on the page, as the user types the email value.
Caveats
This solution was tested in IE 9, IE 11, and Chrome v 33. The context menu paste may not register in some browsers/versions, such as IE 11. "onblur" can be utilized with the same script as below as an additional event to help with this issue, but the script only runs when the cursor leaves the textbox.
Workspace Layout
Components used to prove the concept:
- Form Builder
- Label
- TextBox
- Button
- ImageComponent x 2
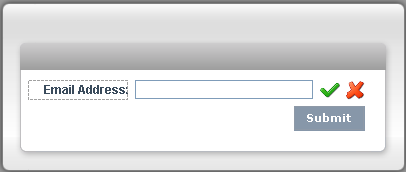
Assumptions
I don't cover the configuration basics here such as input/output variables, field requirements, path connections, etc.
Component Configuration
Configure the Control IDs on the TextBox and 2 ImageComponents.
- TextBox Component ID: EmailAddressText
- ImageComponent 1 Component ID: EmailValid (Green Check)
- ImageComponent 2 Component ID: EmailInvalid (Red X)
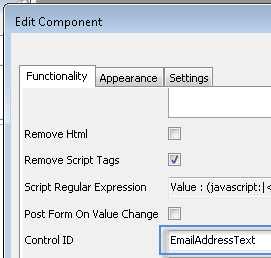
Form Configuration
Right-click on the form itself, and Edit Form.
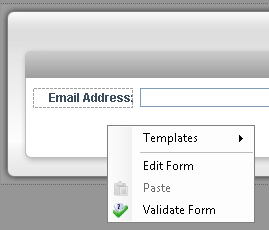
Select the "Behavior" tab, and add a "Body Custom Events" AttributesKeyValuePair event.
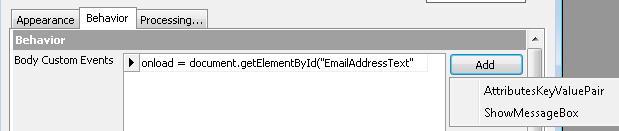
Select the "onload" event, and click the ellipsis for Event Handler.
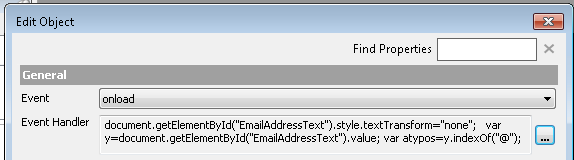
This is where you'll enter the script for the onload validation. This step is taken to validate any existing value that the variable has at page load.
The script:
document.getElementById("EmailAddressText").style.textTransform="none";
var y=document.getElementById("EmailAddressText").value;
var atypos=y.indexOf("@");
var dotypos=y.lastIndexOf(".");
if (atypos<1 || dotypos<atypos+2 || dotypos+2>=y.length)
{
document.getElementById("EmailInvalid").style.visibility="visible";
document.getElementById("EmailValid").style.visibility="hidden";
}
else
{
document.getElementById("EmailValid").style.visibility="visible";
document.getElementById("EmailInvalid").style.visibility="hidden";
}
The javascript I'm using is a mash-up result of searching Google, StackOverflow, and limited personal javascript knowledge. The script first ensures that the textbox does not attempt to capitalize any letters automatically - what the user enters is what is typed in, without any auto-correction or auto-caps. For some reason the workspace I'm using at the moment, whether it was the browser or the workflow code, was auto-capitalizing letters for me. The "style.textTransform="none";" ensures that this does not occur. The script following sets variables for position indices as well as the textbox control we are evaluating. What this translates to is that the email string must:
- Contain only 1 "@" sign, with the "@" sign not being the first character of the string
- Contain at least 1 "." dot, with 1 dot existing after the "@" sign
- Contain a minimum of 2 characters before the end
Source of the email validation javascript: http://www.w3schools.com/js/js_form_validation.asp
TextBox Configuration
This script must also be included in the textbox component in order to check the string as the user types.
Right-click on the textbox component and click "Edit Component".
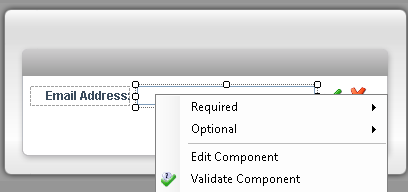
In the "Behavior" section of the "Functionality" tab, Add an AttributesKeyValuePair event for "Custom Events".
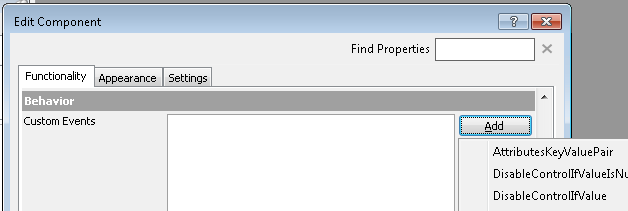
We will need to add 2 separate events, one for "onpropertychange", and another for "onkeyup".
First we will add the "onpropertychange" event. This will fire on events such as browser-based autocomplete, right-click and paste, and ctrl-V.
Select the "onpropertychange" event, and click the ellipsis for Event Handler.
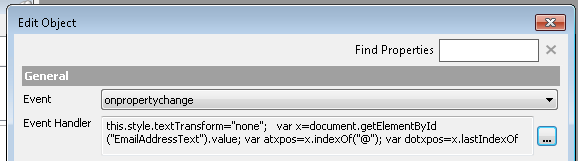
This is where you'll enter the script for the onpropertychange validation.
The script:
this.style.textTransform="none";
var x=document.getElementById("EmailAddressText").value;
var atxpos=x.indexOf("@");
var dotxpos=x.lastIndexOf(".");
if (atxpos<1 || dotxpos<atxpos+2 || dotxpos+2>=x.length)
{
document.getElementById("EmailInvalid").style.visibility="visible";
document.getElementById("EmailValid").style.visibility="hidden";
}
else
{
document.getElementById("EmailValid").style.visibility="visible";
document.getElementById("EmailInvalid").style.visibility="hidden";
}
Next we'll add the "onkeyup" event. This will validate the string after every keypress.
In the "Behavior" section of the "Functionality" tab, Add another AttributesKeyValuePair event for "Custom Events".
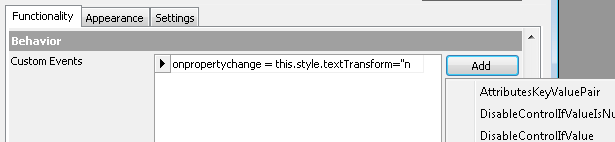
Select the "onkeyup" event, and click the ellipsis for Event Handler.
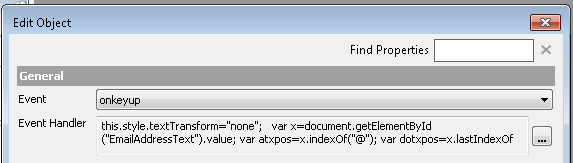
This is where you'll enter the script for the onkeyup validation. It's the same script we used for the onpropertychange event.
The script:
this.style.textTransform="none";
var x=document.getElementById("EmailAddressText").value;
var atxpos=x.indexOf("@");
var dotxpos=x.lastIndexOf(".");
if (atxpos<1 || dotxpos<atxpos+2 || dotxpos+2>=x.length)
{
document.getElementById("EmailInvalid").style.visibility="visible";
document.getElementById("EmailValid").style.visibility="hidden";
}
else
{
document.getElementById("EmailValid").style.visibility="visible";
document.getElementById("EmailInvalid").style.visibility="hidden";
}
Button Configuration
Now we have to ensure that trying to submit a blank or invalid email address is treated as invalid by the submit button.
Right-click on the button component and "Edit Component".
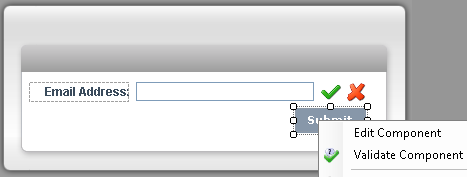
In the "Behavior" section of the "Functionality" tab, Add an event for "Custom Events". "AttributesKeyValuePair" is selected automatically.
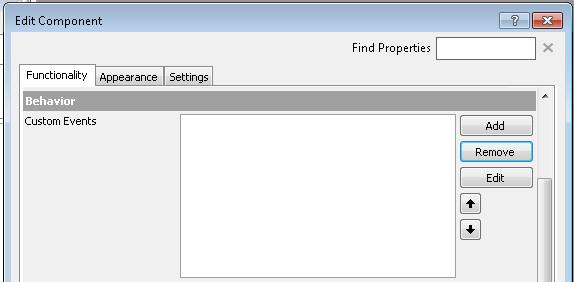
Select the "onclick" event, and click the ellipsis for Event Handler.
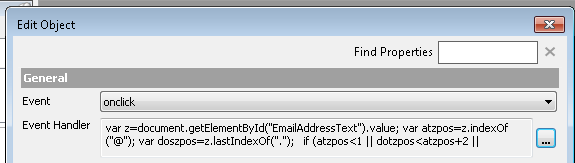
This is where you'll enter the script for the onclick validation. You can edit the Alert to read however you like.
The script:
var z=document.getElementById("EmailAddressText").value;
var atzpos=z.indexOf("@");
var doszpos=z.lastIndexOf(".");
if (atzpos<1 || dotzpos<atzpos+2 || dotzpos+2>=z.length)
{
alert("Email address invalid.");
return false;
}
Final Steps
After all the script events are complete, lay one image component directly over the other. Only one can be visible at a given time, so we want the icon to appear to change in-place. Size the components the way you want and save everything.
Note: The textbox component should be set to "Optional" for the Submit button's requirements. There should be a workflow validation model present to enforce proper content entry as well, in the case that the client-side javascript doesn't apply or doesn't function as intended.
Resources
Attached is an example project with this proof of concept illustrated.